- Published on
How to query a Firestore collection for documents added today
Caveats
This example assumes that each document in your collection has a date field. I'm also using the Firebase Admin SDK since this code is run in a Firebase Function.
I have a date
field on each of the documents in my notes
collection.
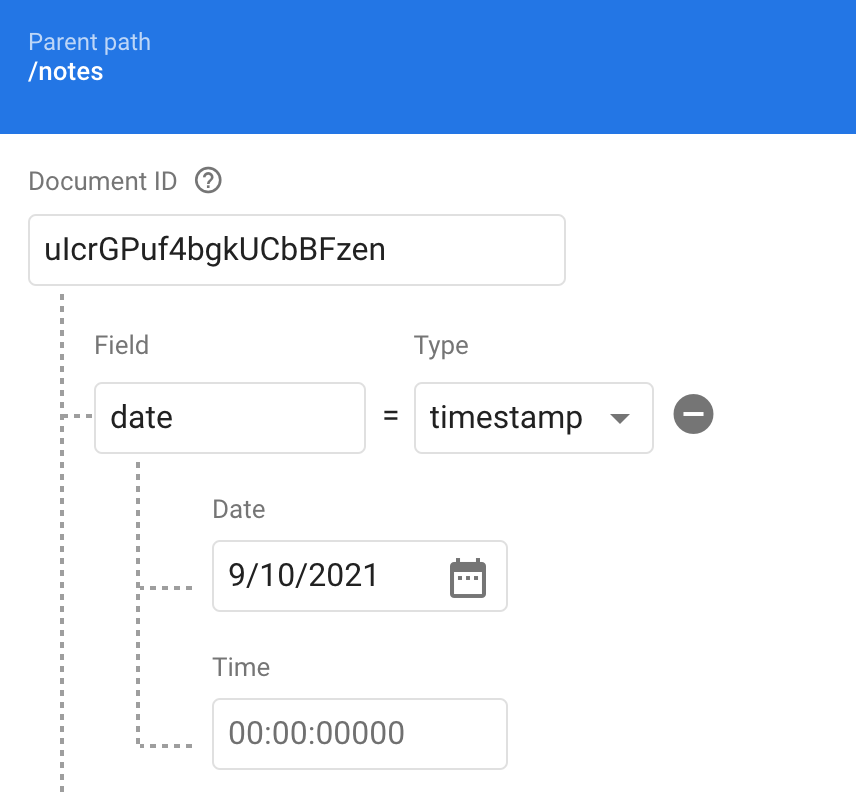
Helper function
In the getStartOfToday
function below, first we get today's date using new Date()
. Then we set it to midnight of today, convert it to a timestamp, and return the timestamp.
function getStartOfToday() {
const now = new Date()
now.setHours(5, 0, 0, 0) // +5 hours for Eastern Time
const timestamp = admin.firestore.Timestamp.fromDate(now)
return timestamp // ex. 1631246400
}
Note: I had to add 5 hours to get EST. You might want to tweak this and test yourself. 🤷♂️
notes
collection
Querying the As you can see below, with the getStartOfToday
function we can run a Firestore query and ask for all documents that have a timestamp greater than the beginning of today. This will return only the documents that have a timestamp from today assuming your date
field is of type Timestamp
. Woot! 🎊
const snapshot = await admin
.firestore()
.collection('notes')
.where('date', '>', getStartOfToday())
.get()
const notes = snapshot.docs.map((doc) => doc.data())
All Together
function getStartOfToday() {
const now = new Date()
now.setHours(5, 0, 0, 0) // +5 hours for Eastern Time
const timestamp = admin.firestore.Timestamp.fromDate(now)
return timestamp // ex. 1631246400
}
const snapshot = await admin
.firestore()
.collection('notes')
.where('date', '>', getStartOfToday())
.get()
const notes = snapshot.docs.map((doc) => doc.data())